MediaWiki extensions and wfLoadExtensionMessages
Upgrading MediaWiki can be a challenging task, especially if you use a lot of extensions. While the core upgrade process usually goes smoothly, it’s rare you can upgrade a major version or two without having to muddle with your collection of extensions. Extensions are bits of code that extend what MediaWiki can do. Only a few are packaged with and maintained alongside MediaWiki itself—the great majority are written by third-party developers. When the MediaWiki API changes, it is up to those developers to update their extension so it works with the new version of MediaWiki. This does not always happen. Take for example one of the more common errors seen on a MediaWiki upgrade since 1.21 was released:
[Tue May 06 11:21:52 2014] [error] [client 12.34.56.78] PHP Fatal error: Call to undefined function wfLoadExtensionMessages() in /home/beckett/mediawiki/extensions/PdfExport/PdfExport.php on line 83, referer: http://test.ziggy.com/wiki/Main_Page
This is because the wfLoadExtensionMessages function, which many extensions use, has been deprecated since MediaWiki version 1.16 and was finally removed in 1.21, resulting in the error seen above. Luckily, this function …
git mediawiki troubleshooting pdf
Drupal Commerce for Fun and Profit
Drupal has for years been known as open source content management system (CMS) for creating easy content-driven websites for any topic. In addition to the CMS, there are a whole host of tools built upon this foundation to extend the features using modules. One module in particular I worked with recently for a client was Drupal Commerce, which helps to augment the CMS with an eCommerce platform.
Within Drupal for normal content items, you would create distinct articles, which have a predefined model of fields that would reflect the different aspects of an article, author, title, tags, etc. While Drupal Commerce uses these same functions, it will also give you the capability to also define SKUs, product categories, and rules and procedures to be carried when adding a particular item to your cart. In much the same way as articles are published to the Drupal taxonomy to allow users to browse through the articles, product categories, and individual products are published, and allow the administrator to customize the layout of how those products would display.
Getting Started with Drupal Commerce
One tool that is extremely helpful on getting a Drupal Commerce setup up for yourself is …
ecommerce drupal cms
Git Workflows That Work
There was a significant blog post some years ago. It introduced a “successful” workflow for using Git. This workflow was named Gitflow. One of the reasons this blog post was significant is that it was the first structured workflow that many developers had been exposed to for using Git. Before Gitflow was introduced, most developers didn’t work with Git like that. And if you remember when it was introduced back in 2010, it created quite a buzz. People praised it as “the” way to work with Git. Some adopted it so quickly and full heartedly that they dismissed any other way to use Git as immature or childish. It became, in a way, a movement.
I start with this little bit of history to talk about the void that was filled by Gitflow. There was clearly something that drew people to it that wasn’t there before. It questioned the way they were working with Git and offered something different that worked “successfully” for someone else. I supposed many developers didn’t have much confidence or strong feelings about their use of Git before they heard of Gitflow. And so they followed someone who clearly did have confidence and strong feelings about a particular workflow. Some of …
git tips
Convert Line Endings of Mac and Windows to Unix in Rails and Test with RSpec
Line endings or newline is a special character(s) to define the end of a line. The line endings special character(s) vary across the operating systems. Let’s take an example, we are developing a Rails feedback application which will be used by a wide range of users. The users might submit the feedback from different operating systems which has different kind of line end character(s). The content should have formatted for standard line endings before storing into backend.
Mostly two special characters used to define the line endings in most of the operating systems.
-
Line Feed (LF) - \n
-
Carriage Return (CR) - \r
The usage of these two special characters for Unix, Mac and Windows are
OS | Characters | Name |
Unix | \n | LF |
Mac | \r | CR |
Windows | \r\n | CRLF |
Note:- \r is the newline character up to Mac OS version 9, after that Mac uses Unix line endings.
It is a developer’s job to convert all kinds of line endings to Unix line ending format to maintain the standard. We can achieve this by a regex pattern replace. The regex pattern should convert \r(Mac) or \r\n(Windows) to \n(Unix).
standard_content = non_standard_content.gsub(/\r\n?/,"\n")
We can see the conversion of line …
ruby rails testing
Liquid Galaxy installation at Westfield State University
This past week End Point installed its newest Liquid Galaxy installation at Westfield State University. The unveiling on April 23rd featured a talk from local glass artist Josh Simpson (whom I have long admired) and a video conference with his wife Cady Coleman, a NASA astronaut. I volunteered to head up the installation effort for two reasons really: First, I was excited to have another Liquid Galaxy in an academic setting, and second, I am originally from western Massachusetts and I couldn’t resist the prospect of some home cooked meals courtesy of my mother.
I arrived at Westfield State to a small army of people who helped me with every aspect of the installation from creative electrical wiring to campus security kindly giving my car a jump. The team at Westfield made this installation a breeze and all the smiling faces made it easy to put in the extra effort to finish in time for the opening. As always I got great remote support from my End Point colleagues during the installation process.
Once the Liquid Galaxy started to take shape the excitement of the students who happened to be passing by was certainly a motivating factor, but the greatest perk was getting to show my …
gis visionport education
Custom plans prepared statements in PostgreSQL 9.2
Someone was having an issue on the #postgresql channel with a query running very fast in psql, but very slow when using DBD::Pg. The reason for this, of course, is that DBD::Pg (and most other clients) uses prepared statements in the background. Because Postgres cannot know in advance what parameters a statement will be called with, it needs to devise the most generic plan possible that will be usable with all potential parameters. This is the primary reason DBD::Pg has the variable pg_server_prepare. By setting that to 0, you can tell DBD::Pg to avoid using prepared statements and thus not incur the “generic plan” penalty. However, that trick will not be needed much longer for most people: version 9.2 of Postgres added a great feature. From the release notes:
Allow the planner to generate custom plans for specific parameter values even when using prepared statements.
Because the original IRC question involved a LIKE clause, let’s use one in our example as well. The system table pg_class makes a nice sample table: it’s available everywhere, and it has a text field that has a basic B-tree index. Before we jump into the prepared statements, let’s …
database dbdpg postgres
RailsConf 2014: My Sketchnote Summary
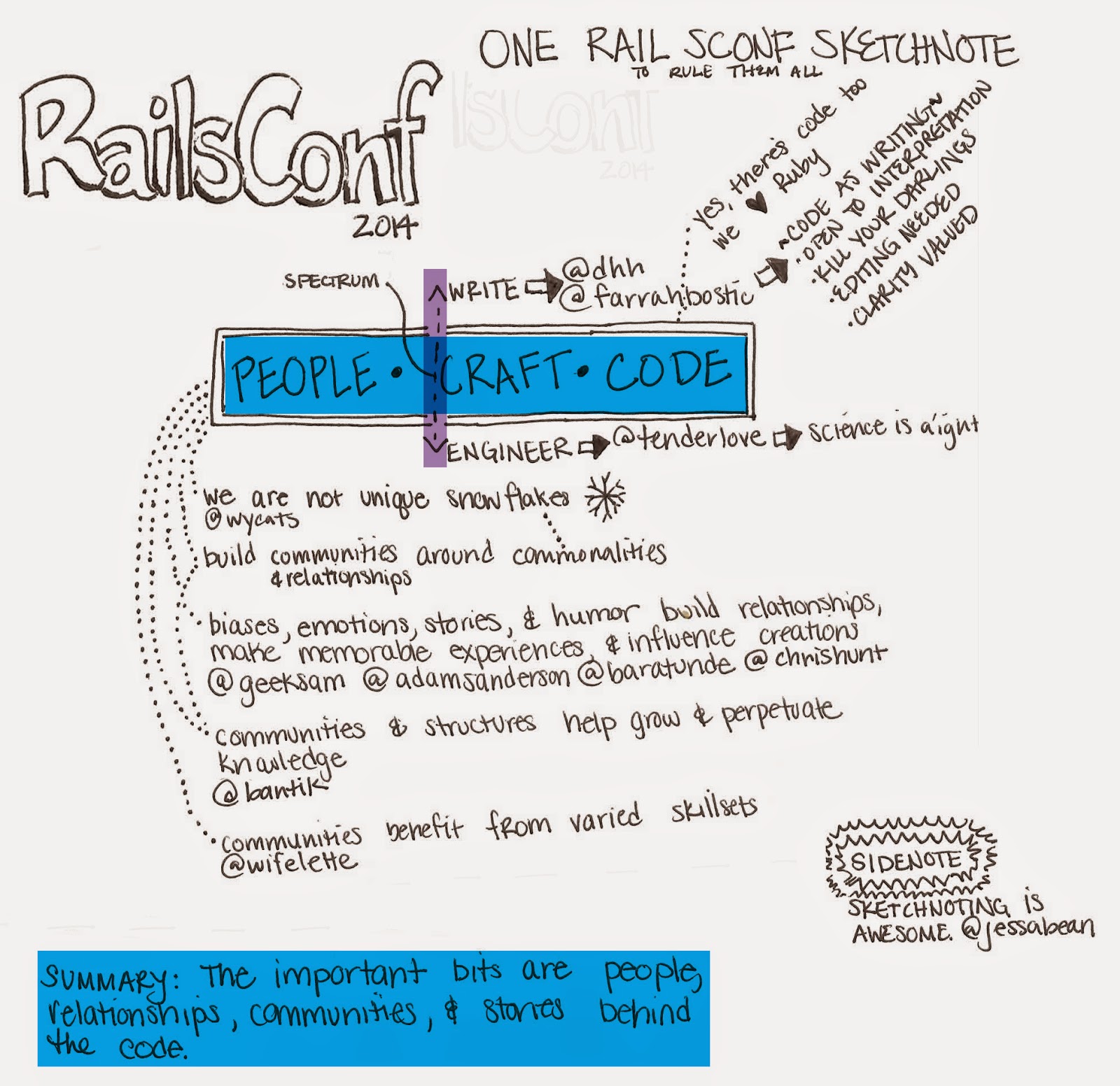
My RailsConf Sketchnote Summary
In the heat of the moment, it’s hard for me to do anything other than regurgitate RailsConf talks into blog articles (case in point: my review of Leah Silber’s talk on building an open source focused company, and my summary of the machine learning workshop at RailsConf). But after a bit of processing and brain-churning, I’ve pieced together the common themes that resonated for me throughout RailsConf, in Sketchnote form!
There was of course a bit of bantering regarding code as “writing” versus code as “engineering” and the arguments supporting both ends of the spectrum. As my fifth RailsConf, I did walk away with some Rails topical knowledge, but the overwhelming theme of many talks I attended was the human element behind coding. People as a part of building communities, sharing commonalities, building relationships and mentorships, being influenced by their own biases and perceptions. Many of my memorable talks of the conference for me had great stories, great humor, and both.
Here’s a list of memorable talks referenced in my sketchnote above if you are interested in checking them out. Not all of the slides have been posted, so I’ll revisit and …
conference ruby rails
Building an Open Source Software-Centric Company at RailsConf 2014
It’s RailsConf 2014 in Chicago and Day 4 (the last day)! Today I attended many good talks. One of them was Building an OSS-Centric Company (and Why You Want To) by Leah Silber. Leah is super passionate and knowledgeable and has good perspective from being involved in many successful open source software companies and projects through the years, including her current work at Tilde on Skylight and Ember.js.
Why Open Source?
First, Leah covered the justification for building a company with a focus on open source (ie how to convince the people that pay you to focus on open source). Here are the bullet points for why open source?:
- Expertise: When you have a company with a focus on open source, you are building the expertise on your own open source tools and you have the available resources as opposed to outsourcing to other companies for expertise. Of course, in my mind, this shouldn’t be a justification for creating an unneeded open source tool, but it’s more of a long-term benefit after your open source projects gain traction.
- Influence and Access: Building a OSS-centric company allows you to sit at the table as stakeholders when it comes to making big decisions about focus, …
conference open-source ruby rails