Debugging Localization in Rails
Rails offers a very extensive library for handling localization using the rails-i18n gem. If you’ve done any localization using Rails, you know that it can be difficult to keep track of every string on your web application that needs translation. During a recent project, I was looking for an easy way to visually see translation issues while browsing through the UI in our application.
Missing Translations
I’ve known for some time that when I was missing a translation for a given text that it would appear in the HTML with a special <span>
tag with the class translation_missing
. So in my global CSS I added the following:
.translation_missing { background-color: red; color: white !important; }
Now when I viewed any page where I had forgotten to add a translation to my en.yml file I could see the text marked in bright red. This served as a great reminder that I needed to add the translation.
I’m an avid watcher of my rails log while I develop. I almost always have a terminal window right next to my browser that is tailing out the log so I can catch any weirdnesses that may crop up. One thing that I thought would be nice is if the log showed any translation issues on the page it …
rails localization
Custom helper subs in Dancer templates
I recently was writing some code using the Dancer Perl web framework, and had a set of HTML links in the template:
<a href="/">Home</a> |
<a href="/contact">Contact</a> |
[etc.]
Since it’s possible this app could be relocated to a different path, say, /something/deeper instead of merely /, I wanted to use Dancer’s handy uri_for() routine to get the full URL, which would include any path relocation. (This concept will be familiar to Interchange 5 users from its [area] and [page] tags.)
The uri_for function isn’t available in templates. The easiest way to cope would be to just use it in my route sub where it works fine, and store the results in the template tokens as strings. But then for any new URL needed I would have to update the route sub and the template, and this feels like a quintessential template concern.
I found this blog post explaining how to add custom functions to be used in templates, and it worked great. Now my template can look like this:
<a href="<% uri_for('/') %>">Home</a> |
<a href="<% uri_for('contact') %>">Contact</a> |
[etc.]
And the URLs are output …
dancer perl
Paper Source Case Study with Google Maps API
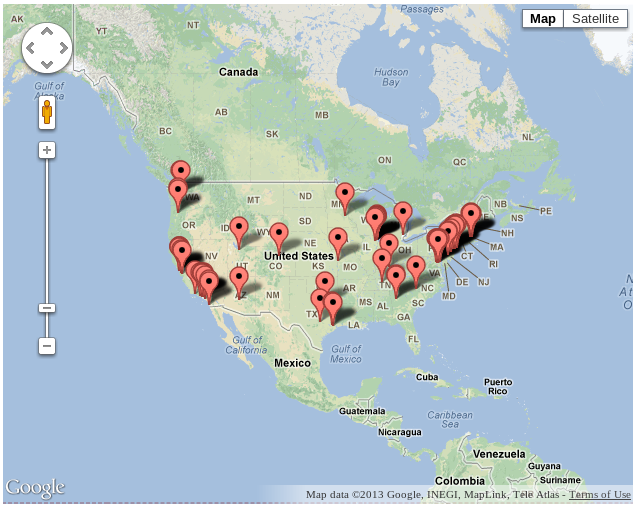
Recently, I’ve been working with the Google Maps API for Paper Source, one of our large Interchange clients with over 40 physical stores throughout the US.
On their website, they had previously been managing static HTML pages for these 40 physical stores to share store information, location, and hours. They wanted to move in the direction of something more dynamic with interactive maps. After doing a bit of research on search options out there, I decided to go with the Google Maps API. This article discusses basic implementation of map rendering, search functionality, as well as interesting edge case behavior.
Basic Map Implementation
In its most simple form, the markup required for adding a basic map with markers is the shown below. Read more at Google Maps Documentation.
HTML
<div id="map"></div>
CSS
#map {
height: 500px;
width: 500px;
}
JavaScript
//mapOptions defined here
var mapOptions = {
center: new google.maps.LatLng(40, -98),
zoom: 3,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
//map is the HTML DOM element ID where it will be rendered
var map = new google.maps.Map(document.getElementById("map"), mapOptions);
//all locations is a …
interchange javascript jquery maps case-study clients
Google Sitemap rapid deployment
I was going to call this “Quick and Dirty Sitemaps”, but “Rapid Deployment” sounds more buzz-word-worthy. This is how to get a Google sitemap up and running quickly, using the Google sitemap generator and the Web Developer Firefox plug-in.
I had occasion to set up a sitemap using the Google sitemap generator for a site recently. Here’s what I did:
Download the generator using the excellent documentation found at the previous link. Unpack it into a convenient location and copy the example_config.xml file to something else, e.g., www.mysite.com_config.xml. Edit the new configuration file and:
- Modify the “base_url” setting to your site;
- Change the “store_into” setting to a file in your site’s document root;
- Add a pointer to a file that will contain your list-of-links, e.g.,
<urllist path="site_urls.txt">
</urllist>
I would locate this in the same path as your new configuration file.
Now, if you don’t already have Web Developer, give yourself a demerit and go install it.
…
Okay, you’ll thank me for that. Now pick a few pages from your site: good choices, depending on your site’s design, are the home page, the sitemap (if you have …
seo
Generating PDF documents in the browser
What you will learn
- How to generate PDF documents in the browser with JavaScript
- How to generate them out of normal HTML
- How to open those PDFs in new windows
- How to render them inline inside the DOM
Introduction
Every once in a while as web developers we face the challenge of providing PDF documents for the data we have persisted in the database.
The usual approach is to:
- generate the document with a library / DSL in the language of the backend
- or—generate normal html view and use a utility like wkhtmltopdf
That works nice, but what if you’re developing an SPA app which only consumes JSON data from its backend? Imagine a scenario when the backend isn’t capable of producing responses other than JSON data. What could you do there?
The solution
Thanks to some very bright folks behind the jsPDF library we have both above mentioned options right inside the browser.
I strongly encourage you to visit their website. There is a nice live coding editor set up which reflects in real time the PDF your code is producing.
Using the DSL
The whole process looks like this:
# 1. create jsPDF object:
doc = new jsPDF()
# 2. put something interesting in there:
doc.setFontSize(22)
doc.text(20, 20 …
javascript pdf
NoSQL benchmark of Cassandra, HBase, MongoDB
We’re excited to have recently worked on an interesting benchmarking project for DataStax, the key company supporting the Cassandra “NoSQL” database for large horizontally-scalable data stores. This was done over the course of about 2 months.
This benchmark compares the performance of MongoDB, HBase, and Cassandra on the widely-used Amazon Web Services (AWS) EC2 cloud instances with local storage in RAID, in configurations ranging from 1-32 database nodes. The software stack included 64-bit Ubuntu 12.04 LTS AMIs, Oracle Java 1.6, and YCSB (Yahoo! Cloud Serving Benchmark) for its lowest-common-denominator NoSQL database performance testing features. Seven different test workloads were used to get a good mix of read, write, modify, and combined scenarios.
Because cloud computing resources are subject to “noisy neighbor” situations of degraded CPU or I/O performance, the tests were run 3 times each on 3 different days, with different EC2 instances to minimize any AWS-related variance.
The project involved some interesting automation challenges for repeatedly spinning up the correct numbers and types of nodes, configuring the node software, running tests, and …
database mongodb nosql performance cassandra
Streaming Live with Red5 Media Server: Two-Way
I already wrote about the basics of publishing and broadcasting with Red5 Media Server. Let’s fast forward to the advanced topics and create a video conference now!
Getting Ready
First, a word about the technology stack: a little bit of Java6/Java EE will be used for the server-side work (Red5 is written in Java), ActionScript2/Adobe Flash CS6 will be the primary tool for the client side development, and OS X Mountain Lion is my operating system.
Red5 Server comes with the set of sample applications that provide the source code for about everything you may want to achieve. The primary challenge is to unleash the power of it, since the samples fall extremely short of documentation! The “fitcDemo” application will serve as a base for all our customization.
Originally I made all the development in Red5 RC 1.0 version where fitcDemo was present. Unfortunately, when I downloaded the latest Red5 1.0.1 release yesterday it was simply not there! The source code was still in the repo, just outdated and not working. Well, I did all the work for Red5 team, so you can just download fitcDemo.war from my repo and drop it into the “webapps” directory of Red5 1.0.1 installation—and you are good! …
java video audio
Data binding in web applications
Ever since JavaScript was introduced the world of web programming has constantly been visited by creative minds, ready to solve burning problems web developers were experiencing.
Navigating through some of the oldest web-dev articles, we still can feel the pain of manipulating the DOM, the-vanilla-way and creating ever so clever hacks to make the code work on all of the web browsers.
Then, the JS-frameworks epidemy started to disseminate. As web developers—we started to have some powerful tools. The productivity of average nerdy Joe raised immediately as he started using Prototype.js, Mootools or jQuery.
Evolution of UI programming for browsers
And so was the start of our global evolution, towards making the Web—our main means of interacting with users. Few with big enough imagination were already seeing HTML, CSS & JS as the future standard of creating user interfaces for data-rich applications. Of course, there were toolkits like ExtJS which aimed at nothing but this—but who would have known back then, that we will be able to build apps for smartphones the way we are building ones for the web?
I have my little observation in life — all hot stuff in the world of technology, …
javascript jquery open-source user-interface