Spree Security Update 2.x.x Error, undefined method ‘assume_from_symbol’ for Money:Class (ActionView::Template::Error)
On March 25, 2014 Spree Commerce posted an announcement that there was a security vulnerability for all Spree 2.x.x versions. As reported on Spree’s website,
“The exploit would require the attacker to randomly guess valid order numbers, but once achieved, the technique would reveal private customer information associated with the order. Credit card details are never stored in Spree and were never at risk by this exploit.”
So, this update is pretty typical and as usual, you should make sure nothing has broken with any changes that were made. I wanted to note a couple of “gotchas” with this most recent update however.
The first of which is probably obvious and really more of a reminder as sometimes people forget. Further, the instructions for this update in the Spree docs don’t mention it, so, as a reminder - after updating be sure to run
$ bundle exec rake railties:install:migrations
$ bundle exec rake db:migrate
Now, here is the tricky one. After updating and installing/running your migrations you may find that you are getting an error:
undefined method `assume_from_symbol' for Money:Class (ActionView::Template::Error)
I have been unable to find …
ecommerce ruby rails security spree update
RailsConf 2014: Highlights from Day One
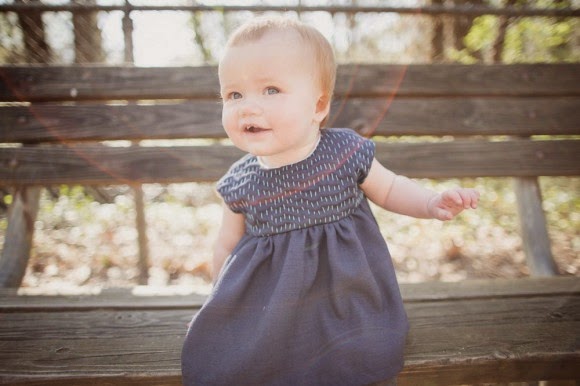
I’m here in Chicago for RailsConf 2014, my fifth RailsConf! This time I’m attending the conference with my sidekick, and of course my sidekick’s babysitter, so I’m having an experience a bit different than previous attendance.
The Bees Knees
One of the first talks I attended today was Saving the World (literally) with Ruby on Rails by Sean Marcia.
Sean works with a Professor at George Mason University who researches bees and dying colonies. Armed with Raspberry Pis powered by solar panels, Sean uses the following technologies to monitor beehive metrics:
- gpio, pi_piper, wiringpi-ruby
- dashing
- Ruby & Sinatra. Sean had previously used Rails but found it a bit too heavyweight and went with a lighter Sinatra app.
- 3 Cronjobs to record data
- passenger, isc-dhcp-server, hostapd, iw for server configuration
Hive temperature, hive weight, outside temperature, and humidity are monitored in hopes of collecting metrics for identifying collapsing hives. The project is quite young, but the hope is to collect more metrics (e.g. gas permeability) and more actionable data as time goes on.
Views as Objects
Another interesting and relevant talk I attended today was …
conference ruby rails
Chrome, onmousemove, and MediaWiki JavaScript
tl;dr: avoid using onmousemove events with Google Chrome.
I recently fielded a complaint about not being able to select text with the mouse on a wiki running the MediaWiki software. After some troubleshooting and research, I narrowed the problem down to a bug in the Chrome browser regarding the onmousemove event. The solution in this case was to tweak JavaScript to use onmouseover instead of onmousemove.
The first step in troubleshooting is to duplicate the problem. In this case, the page worked fine for me in Firefox, so I tried using the same browser as the reporter: Chrome. Sure enough, I could no longer hold down the mouse button and select text on the page. Now that the browser was implicated, it was time to see what it was about this page that caused the problem.
It seemed fairly unlikely that something like this would go unfixed if it was happening on the flagship MediaWiki site, Wikipedia. Sure enough, that site worked fine, I could select the text with no problem. Testing some other random sites showed no problems either. Some googling indicated others had similar problems with Chrome, and gave a bunch of workarounds for selecting the …
browsers chrome mediawiki troubleshooting
Firefox, Input (type=button), and Line-Height
I recently discovered a discrepancy in the way Firefox treats inputs with a line-height style defined and how other browsers handle the same styling rule. Specifically, Firefox completely ignores it.
This behavior seemed odd enough to me that I did some Googling to determine if this was recently introduced, a long standing issue, or something I was just doing wrong. I found some interesting discussions on the issue. Several of the search results used the word “bug” in the title though it appears to be more of a deliberate (though possibly outdated) “feature” instead. Along with the discussions, I also came across a couple of suggestions for a solution.
First of all, I was able to locate a simple enough explanation of what’s causing the behavior. As Rob Glazebrook explains:
Basically, Firefox is setting the line-height to “normal” on buttons and is enforcing this decision with an !important declaration.” and, “browser-defined !important rules cannot be over-ruled by author-defined !important rules. This rule cannot be overruled by a CSS file, an inline style—anything.
Good news is I can stop experimenting hoping for different results.
I also located a Bugzilla ticket opened in …
browsers
Piggybak: Upgrade to Rails 4.1.0
Piggybak and gems available in the demo (piggybak_variants, piggybak_giftcerts, piggybak_coupons, piggybak_bundle_discounts, piggybak_taxonomy) have been updated to Rails 4.1.0, Ruby 2.1.1 via Piggybak version gem 0.7.1. Interested in the technical details of the upgrade? Here are some fine points:
- Dependencies were refactored so that the parent Rails app controls the Rails dependency only. There was a bit of redundancy in the various plugin gemspec dependencies. This has been cleaned up so the parent Rails app shall be the canonical reference to the Rails version used in the application.
- Modified use of assets which require “//= require piggybak/piggybak-application” to be added to the assets moving forward. There have been several observed issues with precompling and asset behavior, so I simplified this by requiring this require to be added to the main Rails application.js for now. The engine file is supposed to have a way around this, but it has not behaved as expected, specifically on unique deployment architectures (e.g. Heroku). Patches welcome to address this.
- Tables migrated to namespaced tables, e.g. “orders” migrated to “piggybak_orders”. This is how namespaced engine …
piggybak rails ecommerce
jQuery Content Replacement with AJAX
This is not a huge breakthrough, but it colored in some gaps in my knowledge so I thought I would share.
Let’s say you have a product flypage for a widget that comes in several colors. Other than some of the descriptive text, and maybe a hidden field for use in ordering one color instead of another, all the pages look the same. So your page looks like this (extremely simplified):
... a lot of boilerplate ...
<form id="order_item">
<input name="sku" type="hidden" value="WDGT-001-RED"/>
<input type="hidden"/>
</form>
... a lot more boilerplate ...
Probably the page is generated into a template based on a parameter or path segment:
http://.../app/product/WDGT-001-RED
What we’re going to add is a quick-and-dirty way of having your page rewrite itself on the fly with just the bits that change when you select a different version (or variant) of the same product. E.g.,
<select name="sku">
<option value="WDGT-001-RED">Cinnamon Surprise</option>
<option value="WDGT-002-BLK">Midnight Delight</option>
<option value="WDGT-003-YLW">Banana Rama</option>
</ …
javascript jquery html
SPF, DKIM and DMARC brief explanation and best practices
Spam mail messages have been a plague since the Internet became popular and they kept growing more and more as the number of devices and people connected grew. Despite the numerous attempts of creation of anti-spam tools, there’s still a fairly high number of unwanted messages sent every day.
Luckily it seems that lately something is changing with the adoption of three (relatively) new tools which are starting to be widely used: SPF, DKIM and DMARC. Let’s have a quick look at each of these tools and what they achieve.
What are SPF, DKIM and DMARC
SPF (Sender Policy Framework) is a DNS text entry which shows a list of servers that should be considered allowed to send mail for a specific domain. Incidentally the fact that SPF is a DNS entry can also considered a way to enforce the fact that the list is authoritative for the domain, since the owners/administrators are the only people allowed to add/change that main domain zone.
DKIM (DomainKeys Identified Mail) should be instead considered a method to verify that the messages’ content are trustworthy, meaning that they weren’t changed from the moment the message left the initial mail server. This additional layer of trustability is …
Speeding Up Saving Millions of ORM Objects in PostgreSQL
The Problem
Sometimes you need to generate sample data, like random data for tests. Sometimes you need to generate it with huge amount of code you have in your ORM mappings, just because an architect decided that all the logic needs to be stored in the ORM, and the database should be just a dummy data container. The real reason is not important—the problem is: let’s generate lots of, millions of rows, for a sample table from ORM mappings.
Sometimes the data is read from a file, but due to business logic kept in ORM, you need to load the data from file to ORM and then save the millions of ORM objects to database.
This can be done in many different ways, but here I will concentrate on making that as fast as possible.
I will use PostgreSQL and SQLAlchemy (with psycopg2) for ORM, so all the code will be implemented in Python. I will create a couple of functions, each implementing another solution for saving the data to the database, and I will test them using 10k and 100k of generated ORM objects.
Sample Table
The table I used is quite simple, just a simplified blog post:
CREATE TABLE posts (
id SERIAL PRIMARY KEY,
title TEXT NOT NULL,
body TEXT NOT NULL,
payload TEXT NOT NULL …
performance postgres python