Keeping Your Apps Neat & Tidy With RequireJS
RequireJS is a very handy tool for loading files and modules in JavaScript. A short time ago I used it to add a feature to Whiskey Militia that promoted a new section of the site. By developing the feature as a RequireJS module, I was able to keep all of its JavaScript, HTML and CSS files neatly organized. Another benefit to this approach was the ability to turn the new feature “on” or “off” on the site by editing a single line of code. In this post I’ll run through a similar example to demonstrate how you could use RequireJS to improve your next project.
File Structure
The following is the file structure I used for this project:
├── index.html
└── scripts
├── main.js
├── my
│ ├── module.js
│ ├── styles.css
│ └── template.html
├── require-jquery.js
├── requirejs.mustache.js
└── text.js
The dependencies included RequireJS bundled together with jQuery, mustache.js for templates and the RequireJS text plugin to include my HTML template file.
Configuration
RequireJS is included in the page with a script tag and the data-main attribute is used to specify additional files to load. In this case “scripts/main” tells RequireJS to load the main.js file that resides in the scripts directory. Require will load the specified files asynchronously. This is what index.html looks like:
<!DOCTYPE html><br/><html><br/><head><br/> <title>RequireJS Example</title><br/></head><br/><body><br/> <h1>RequireJS Example</h1><br/> <!-- This is a special version of jQuery with RequireJS built-in --><br/> <script data-main="scripts/main" src="scripts/require-jquery.js"></script><br/></body><br/></html><br/>
I was a little skeptical of this approach working on older versions of Internet Explorer so I tested it quickly with IE6 and confirmed that it did indeed work just fine.
Creating a Module
With this in place, we can create our module. The module definition begins with an array of dependencies:
define([
"require",
"jquery",
"requirejs.mustache",
"text!my/template.html"
],
This module depends on require, jQuery, mustache, and our mustache template. Next is the function declaration where our module’s code will live. The arguments specified allow us to map variable names to the dependencies listed earlier:
function(require, $, mustache, html) { ... }
In this case we’re mapping the $ to jQuery, mustache to requirejs.mustache and, html to our template file.
Inside the module we’re using Require’s .toUrl() function to grab a URL for our stylesheet. While it is possible to load CSS files asynchronously just like the other dependencies, there are some issues that arise that are specific to CSS files. For our purposes it will be safer to just add a element to the document like so:
var cssUrl = require.toUrl("./styles.css");
$('head').append($('<link/>',
{ rel: "stylesheet", media: "all", type: "text/css", href: cssUrl }));
Next, we define a view with some data for our Mustache template and render it.
var view = {
products: [
{ name: "Apples", price: 1.29, unit: 'lb' },
{ name: "Oranges", price: 1.49, unit: 'lb'},
{ name: "Kiwis", price: 0.33, unit: 'each' }
],
soldByPound: function(){
return (this['unit'] === 'lb') ? true : false;
},
soldByEach: function() {
return (this['unit'] === 'each') ? true : false;
}
}
// render the Mustache template
var output = mustache.render(html, view);
// append to the HTML document
$('body').append(output);
});
The Template
I really like this approach because it allows me to keep my HTML, CSS and JavaScript separate and also lets me write my templates in HTML instead of long, messy JavaScript strings. This is what our template looks like:
<ul class="hot-products"><br/> {{#products}}<br/> <li class="product"><br/> {{name}}: ${{price}} {{#soldByEach}}each{{/soldByEach}}{{#soldByPound}}per lb{{/soldByPound}}<br/> </li><br/> {{/products}}<br/></ul><br/>
Including the Module
To include our new module in the page, we simply add it to our main.js file:
require(["jquery", "my/module"], function($, module) {
// jQuery and my/module have been loaded.
$(function() {
});
});
When we view our page, we see that the template was was rendered and appended to the document:
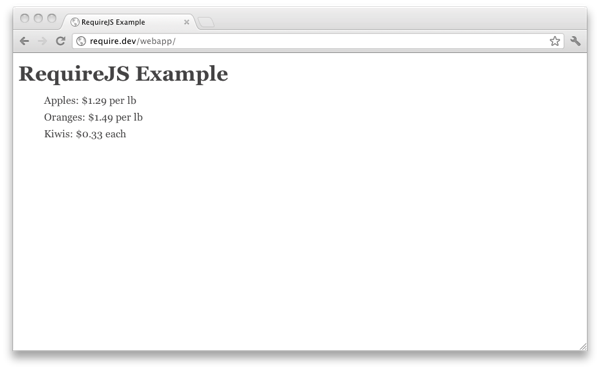
Optimizing Your Code With The r.js Optimizer
One disadvantage of keeping everything separate and using modules in this way is that it adds to the number of HTTP requests on the page. We can combat this by using the the RequireJS Optimizer. The r.js script can be used a part of a build process and runs on both node.js and Rhino. The Optimizer script can minify some or all of your dependencies with UglifyJS or Google’s Closure Compiler and will concatenate everything into a single JavaScript file to improve performance. By following the documentation I was able to create a simple build script for my project and build the project with the following command:
node ../../r.js -o app.build.js
This executes the app.build.js script with Node. We can compare the development and built versions of the project with the Network tab in Chrome’s excellent Developer Tools.
Development Version:
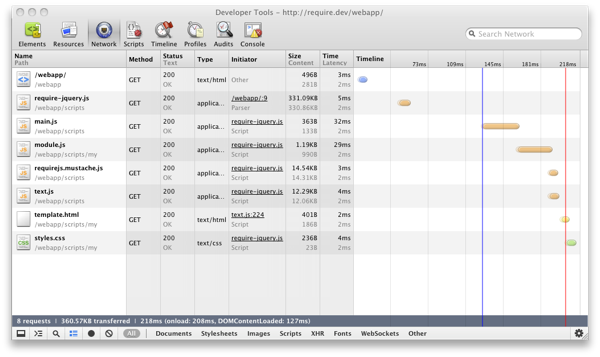
Optimized with the RequireJS r.js optmizer:
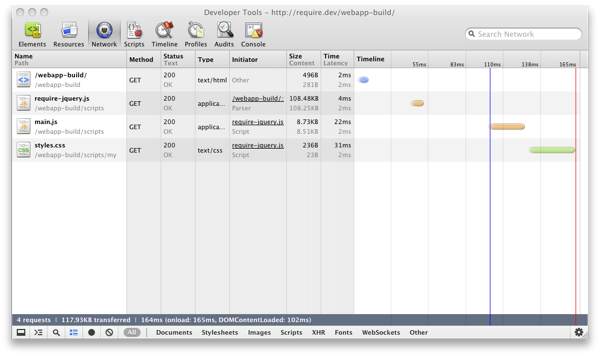
It’s great to be able to go from 8 HTTP requests and 360 KB in development mode to 4 HTTP requests and ~118 KB after by running a simple command with Node! I hope this post has been helpful and that you’ll check out RequireJS on your next project.
css javascript jquery open-source tools
Comments